App Development Curriculum
1. Starting with Flutter
-
Setting up flutter with Android Studio.
-
Understand Flutter widget tree and pre made widget for interface.
-
Incorporate image and text widget.
-
Incorporate flutter icons for Android and IOS.
-
Incorporate explict icons and image assets.
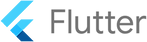
2. Creating UI with Flutter
-
Introduction to bulid method.
-
Creating multiple widgets and its incorporation.
-
Use the pubspec.yml file to incorporate dependencies,custom assets and fonts
-
Use of layout widgets such as columns, Rows, Container, Cards Button, Text field, Forms and others etc.
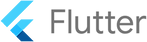
3. Building Apps with state
-
Understand the differences between stateful and stateless widget and when they should each be used.
-
Understand how callbacks can be used defect user interaction in buttons widgets.
-
Understand the declarative style of UI programming and how flutter widgets react to state changes.
-
Learn to import dart libraries to incorporate additional functionality.
-
Learn about how variable, data types and functions work in Dart 03 Structuring Flutter
-
Apps Build flexible layouts using the flutter expanded widget.
-
Understand the relationship between setstate.state objects and stateful widget.
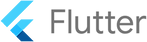
4. Structuring Flutter Apps
-
Learn about how lists and conditional work in dart.
-
Learn about classes and objects in dart and how it apply to widget flutter.
-
Understand the objects Oritented dart and how to apply the fundmentals of OOPs to restructing a flutter app.
-
Learn to use dart constructors to create customisable flutter widgets.
-
Apply common mobile design pattern to structure flutter apps.
-
Learn about structuring and organising flutter apps.
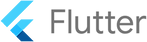
5. Creating UI with Flutter
-
Customise app with themes widget.
-
Refactoring widgets by extracting them as separate widgets classess
-
Learn about Dart annotations and modifiers.
-
Understand the immutability of stateless and stateful widgets and how the screen is updated with the build method.
-
Create custom flutter widgets by combing smaller widgets.
-
Learn about the difference between final and const in dart.
-
Learn about the maps, enums and the ternay operator in dart.
-
Understand that functions are the first class objects in dart and how functions can be passed around as arugments.
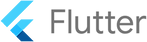
6. Using Backend data with
flutter app
-
Learn about asynchronous programming in dart and understand how to asyn/await and flutter API.
-
Understand Stateful widget lifecycle methods.
-
Handling expections in dart with try/catch and throw.
-
Use Dart null aware operators to prevent app crashes.
-
Getting location data from both ios and android
-
Using the http package to perform networking and get live data from open APIS.
-
Understanding how to parse JSON data using the dart: convert library.
-
Understanding how to pass data to state objects via the stateful widgets.
-
Use the textfield widget to take user input.
-
Understand how to pass data backwards using the navigator
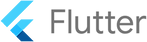
7. Integrating flutter apps with firebase
-
Incorporate Firebase Cloud firestore into your flutter apps.
-
Implement authentication in your flutter apps with the firebase auth package.
-
Build a scrolling listview widget to learn how flutter creates and destroys.
-
Using push notification Using FCM
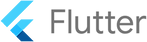